Week 04 project 1
Thanks my group Yuan Fang and Cynthia Ye. I was responsible for the programming and wiring in this project. Thanks to my teammates for their encouragement and patience when I encountered difficulties. It was really a difficult project for me, but at the same time I gained a lot of new knowledge.
After much effort I still have two problems to solve.
1. how to make the sensor and speaker work at the same time? I already know that I can use millis() to store the time without using delay(). But I still don't know how to use millis to play music while using for loop.
2. My line and code seem to be fine, but Fan has sometimes not worked. After restarting several times it will start working again. So I'm not sure if it's a soldering problem or if the Arduino is loaded with too much stuff that causes slow startup sometimes, or maybe there's something else? The fan I am using has three wires and I only connected two of them. Is it because of this that it sometimes does not work
This was our initial idea.
Ultrasonic sensors measure the distance to control the brightness of the bulb and the speed of the fan. As the spectator's hand gets closer to the ball, the brighter the bulb will be and the fan will spin faster, blowing the feathers up.
Basically, this device will have two states.
1. Starting state, the LED is breathing and the fan is not moving
2. When the user is less than 40 cm away, the fan starts to rotate and the light bulb starts to become bright
It was nice to imagine, but in fact we encountered many problems.

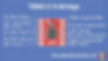
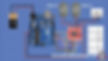
The code of motor
// Motor A int pwmA = 5;
int in1A = 3;
int in2A = 4;
void setup()
{
// Set all the motor control pins to outputs
pinMode(pwmA, OUTPUT);
pinMode(pwmB, OUTPUT);
pinMode(in1A, OUTPUT);
pinMode(in2A, OUTPUT);
}
void loop() {
// Set Motor A forward
digitalWrite(in1A, HIGH);
digitalWrite(in2A, LOW);
//use pwmA control the motor
}
The positive and negative poles of the motor need to be set up in Setup (to establish the direction of rotation of the motor), VM connected to a suitable external power supply.
At first I used a 9V power supply as an external power source(VM & GND), but the motor turned very slowly. Then I realized that the battery was dead. So it is important to test with a multimeter before use.
On Wednesday morning, when I thought our project should have been almost finished. Because I hadn't gotten to the floor yet, my teammate made the mistake of connecting the external power supply directly to the Arduino during testing. The Arduino and the power supply both burned up, and maybe all the accessories as well. I don't know because I still have a class before pcom. I feel very very very very frustrated, but this also proves again the importance of the motor driver, which protects the Arduino very well.
Having something shorted out seems common and I can totally understand that. It's just that this happened before the presentation and it made me really frustrated. Because we did work on it for a long time.
Problem 1 Motor driver
I used my own previously purchased equipment for testing (motor and Potentiometer) before the equipment we purchased arrived. Thanks to Resistant Lulu for telling me that I should use the motor driver to drive the motor/Fan and for showing me the datasheet of the motor driver, very useful!
Problem 2 PWM pin (~)
When I wrote the above code and connected the motor, I found that the motor state change is not continuous, only on and off. I discussed this problem with Yuan Fang and we tried the simplest LAB before, but it still failed. Analog data was not transmitted and the motor/LED was always on and off.
Thanks to our senior, Yifei Gao, he was very patient and helped us to check the circuit. He pointed out that my problem was not using the pin with "~".
I think I will always remember after this failure that when I want to use analogWrite, I will make sure that Pin comes with ~
When I transferred Pin to 3, the lamp and ultrasonic sensor worked in interaction.
Problem 3 Fan threshold
Just when I thought everything should become easy, I met a new difficulty. I found that everything was fine when Fan dropped from 255 to 0, but when Fan stopped and had to start slowly (below 140), Fan didn't work. I need to push the fan a little bit for it to turn. I also cut my finger as a result, hard lesson learned. :(
I shared the difficulties I was having with my teammates again, but we still didn't come to a discussion. I guessed that it wasn't starting too slowly resulting in not enough voltage. I tried to make some changes to get the Map value to go from 130 to 255 instead of 0 to 255. so that the fan would never stop completely. This way it worked successfully.
if(distance<50){
sensorValue=map(distance,0,50,255,130);
analogWrite(pwmA,sensorValue);
}else{
sensorValue=130;
analogWrite(pwmA,sensorValue);
}
I tried changing 130 to 120 again, but it stopped once again. Although I understand how to solve it, I still want to know the reason. Since Tom's office hours were full, we had an appointment with David. He meticulously told us that motor would have a THRESHOLD and suggested that the states we would try to interact with directly were OFF and ON.
Problem 4 Electric fan with three wires
Because the fan we bought was too small (should have paid attention to the power), so I found two larger fans from the junk pile. But they have three wires, so I guess one should be a signal wire? Since there are only two connectors on the motor driver, I gave up on connecting the third cable. (My fans sometimes don't work, is that because I didn't link the third wire? )Before the motor does not distinguish between positive and negative poles, but this big fan has to pay attention to the positive and negative poles to work properly.
Problem 5 LED breathing and sensor checking
In our design, the device should be breathing in the initial state without detecting people approaching. the LED will gradually light up and fade down. I started out using a for loop.
for (int a=0; a<=255;a++)
{
analogWrite(ledpin,a);
delay(8);
}
for (int a=255; a>=0;a--)
{
analogWrite(ledpin,a);
delay(10);
}
The LED works well and beautifully, but the problem is that the sensor does not make the next detection until the end of the two For loop.The sensor should be detecting all the time, not working only every few seconds.
So I asked Lulu again. She patiently told me I should use millis() to solve the problem and explained how millis works. I looked up for a long time and read a lot of cases, but I still didn't know how to use millis in a state where both IF judgments and for loops are performed.
Finally I thought of trigonometric functions, and I used the Sin function.
time=millis();
int fadein=128+127*sin(2*PI/fade*time);
analogWrite(ledPin1,fadein);
analogWrite(ledPin2,fadein);
Problem 6 speaker play music and sensor check
Similarly, when using speaker to play music, you will also encounter the above problem. Lulu also said it would be very complicated. Plus, if we follow the previous plan, the ultrasonic sensor should be placed on the side of the ball, which is more difficult to install. I thought we might be able to change the plan. So I suggested that maybe we could turn the magic ball into a magic detector. People come to the magic ball, the magic ball willrandom a value from 160-255, when the value is greater than 200, speaker will play the Harry Potter theme song, the value is less than 200, speaker will play the Mario theme song (muggle music, lol). So that when speaker plays music, the sensor happens not to detect it. This gives the user a time to change person, perfect!
My teammates agreed with the idea, so I started rewriting the code. Many thanks to Yuan Fang, who wrote the code for the Harry Potter theme song, which reduced my workload a lot.!
But then I ran into the problem that wizards and muggles make little difference in the speed of fan rotation sometimes. (For example, 190 and 200).
//wizard or mugle
if(sensorValue>=200){
analogWrite(pwmA,sensorValue);
analogWrite(pwmB,sensorValue);
analogWrite(ledPin1,sensorValue);
analogWrite(ledPin2,sensorValue);
for (int thisNote = 0; thisNote < 30; thisNote++) {
int noteDuration = 1500/noteDurations[thisNote];
tone(8, melody[thisNote],noteDuration);
delay(noteDuration +30);
noTone(8);
}
}else {
sensorValue=160;
analogWrite(pwmA,sensorValue);
analogWrite(pwmB,sensorValue);
analogWrite(ledPin1,sensorValue);
for (int thisNote = 0; thisNote < 60; thisNote++) {
int noteDuration = 1000/noteDurationsMuggle[thisNote];
tone(8, melodyMuggle[thisNote],noteDuration);
//pause for the note's duration plus 30 ms:
delay(noteDuration +30);
noTone(8);
}
}
Problem 7 Feathers and fans
Sorry I really don't have time to finish my blog, I will make up for it as soon as I can this week. There are so many things I want to document about this project